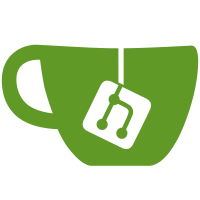
The language for this was very strange, so I rewrote it in a more standard format. Instead of printing things across three functions, I consolidated into one. I also opted for the more standard "WARNING:" instead of "***", which I've not seen before.
105 lines
3.7 KiB
Python
Executable File
105 lines
3.7 KiB
Python
Executable File
#!/usr/bin/python2
|
|
# -*- encoding: utf8 -*-
|
|
#
|
|
# The Qubes OS Project, http://www.qubes-os.org
|
|
#
|
|
# Copyright (C) 2010 Joanna Rutkowska <joanna@invisiblethingslab.com>
|
|
#
|
|
# This program is free software; you can redistribute it and/or
|
|
# modify it under the terms of the GNU General Public License
|
|
# as published by the Free Software Foundation; either version 2
|
|
# of the License, or (at your option) any later version.
|
|
#
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with this program; if not, write to the Free Software
|
|
# Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301,
|
|
# USA.
|
|
|
|
import os
|
|
import sys
|
|
from optparse import OptionParser
|
|
|
|
from qubes.qubes import QubesVmCollection
|
|
|
|
|
|
def main():
|
|
usage = "usage: %prog [options] <src-name> <new-name>\n"\
|
|
"Clones an existing VM by copying all its disk files"
|
|
|
|
parser = OptionParser(usage)
|
|
parser.add_option("-q", "--quiet", action="store_false", dest="verbose",
|
|
default=True)
|
|
parser.add_option("-p", "--path", dest="dir_path",
|
|
help="Specify path to the template directory")
|
|
parser.add_option("--force-root", action="store_true", dest="force_root",
|
|
default=False,
|
|
help="Force to run, even with root privileges")
|
|
parser.add_option("-P", "--pool", dest="pool_name",
|
|
help="Specify in to which storage pool to clone")
|
|
|
|
(options, args) = parser.parse_args()
|
|
if (len(args) != 2):
|
|
parser.error(
|
|
"You must specify at least the src and dst TemplateVM names!")
|
|
srcname = args[0]
|
|
dstname = args[1]
|
|
|
|
if hasattr(os, "geteuid") and os.geteuid() == 0:
|
|
if not options.force_root:
|
|
print >> sys.stderr, "WARNING: Running this tool as root can lead to" + \
|
|
" permission problems and is strongly discouraged. Please" + \
|
|
" retry as an unprivileged user or use --force-root to" + \
|
|
" continue anyway."
|
|
exit(1)
|
|
|
|
qvm_collection = QubesVmCollection()
|
|
qvm_collection.lock_db_for_writing()
|
|
qvm_collection.load()
|
|
|
|
src_vm = qvm_collection.get_vm_by_name(srcname)
|
|
if src_vm is None:
|
|
print >> sys.stderr, \
|
|
"ERROR: A VM with the name '{0}' does not exist in the system." \
|
|
.format(srcname)
|
|
exit(1)
|
|
|
|
if options.pool_name is None:
|
|
pool_name = src_vm.pool_name
|
|
else:
|
|
pool_name = options.pool_name
|
|
|
|
if qvm_collection.get_vm_by_name(dstname) is not None:
|
|
print >> sys.stderr, \
|
|
"ERROR: A VM with the name '{0}' already exists in the system." \
|
|
.format(dstname)
|
|
exit(1)
|
|
|
|
if src_vm.is_disposablevm():
|
|
print >> sys.stderr, "ERROR: Clone not supported for this type of VM"
|
|
exit(1)
|
|
|
|
dst_vm = qvm_collection.add_new_vm(src_vm.__class__.__name__,
|
|
name=dstname, template=src_vm.template,
|
|
pool_name=pool_name,
|
|
dir_path=options.dir_path,
|
|
installed_by_rpm=False)
|
|
|
|
try:
|
|
dst_vm.clone_attrs(src_vm)
|
|
dst_vm.clone_disk_files(src_vm=src_vm, verbose=options.verbose)
|
|
except (IOError, OSError) as err:
|
|
print >> sys.stderr, "ERROR: {0}".format(err)
|
|
qvm_collection.pop(dst_vm.qid)
|
|
dst_vm.remove_from_disk()
|
|
exit(1)
|
|
|
|
qvm_collection.save()
|
|
qvm_collection.unlock_db()
|
|
|
|
main()
|