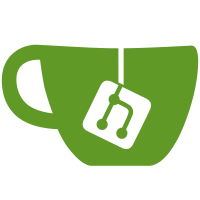
qvm-clock-sync fails with a python subprocess error on line 43 when the `date ... -s ...` fails due to insufficient privileges to set the date. This commit checks to see if the program has effective UID root before attempting to set the date.
51 lines
1.7 KiB
Python
Executable File
51 lines
1.7 KiB
Python
Executable File
#!/usr/bin/python3
|
|
# -*- encoding: utf8 -*-
|
|
#
|
|
# The Qubes OS Project, http://www.qubes-os.org
|
|
#
|
|
# Copyright (C) 2010 Marek Marczykowski <marmarek@invisiblethingslab.com>
|
|
#
|
|
# This library is free software; you can redistribute it and/or
|
|
# modify it under the terms of the GNU Lesser General Public
|
|
# License as published by the Free Software Foundation; either
|
|
# version 2.1 of the License, or (at your option) any later version.
|
|
#
|
|
# This library is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
|
|
# Lesser General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU Lesser General Public
|
|
# License along with this library; if not, see <https://www.gnu.org/licenses/>.
|
|
#
|
|
#
|
|
import sys
|
|
import os
|
|
import re
|
|
import subprocess
|
|
from qubesadmin import Qubes
|
|
|
|
def main():
|
|
if os.geteuid() != 0:
|
|
sys.stderr.write('This program must be run as root to set the date.')
|
|
sys.exit(1)
|
|
app = Qubes()
|
|
clockvm = app.clockvm
|
|
|
|
p = clockvm.run_service('qubes.GetDate')
|
|
untrusted_date_out = p.stdout.read(25).decode('ascii', errors='strict')
|
|
untrusted_date_out = untrusted_date_out.strip()
|
|
|
|
if not re.match(r'^\d{4}-\d{2}-\d{2}T\d{2}:\d{2}:\d{2}\+00:?00$', untrusted_date_out):
|
|
sys.stderr.write('Invalid date received, aborting!\n')
|
|
sys.exit(1)
|
|
date_out = untrusted_date_out
|
|
subprocess.check_call(['date', '-u', '-Iseconds', '-s', date_out],
|
|
stdout=subprocess.DEVNULL)
|
|
subprocess.check_call(['hwclock', '--systohc'],
|
|
stdout=subprocess.DEVNULL)
|
|
|
|
if __name__ == '__main__':
|
|
main()
|
|
|