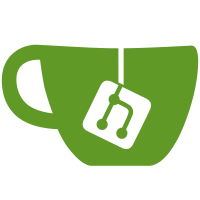
This way it will work independently from where qrexec-policy tool will be called (in most cases - from a system service, as root). This is also very similar architecture to what we'll need when moving to GUI domain - there GUI part will also be separated from policy evaluation logic. QubesOS/qubes-issues#910
71 lines
2.2 KiB
Python
71 lines
2.2 KiB
Python
# -*- encoding: utf8 -*-
|
|
#
|
|
# The Qubes OS Project, http://www.qubes-os.org
|
|
#
|
|
# Copyright (C) 2017 Marek Marczykowski-Górecki
|
|
# <marmarek@invisiblethingslab.com>
|
|
#
|
|
# This program is free software; you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation; either version 2 of the License, or
|
|
# (at your option) any later version.
|
|
#
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License along
|
|
# with this program; if not, see <http://www.gnu.org/licenses/>.
|
|
|
|
|
|
''' Agent running in user session, responsible for asking the user about policy
|
|
decisions.'''
|
|
|
|
import pydbus
|
|
import gi
|
|
gi.require_version('Gtk', '3.0')
|
|
from gi.repository import GLib
|
|
|
|
import qubespolicy.rpcconfirmation
|
|
|
|
class PolicyAgent(object):
|
|
dbus = """
|
|
<node>
|
|
<interface name='org.qubesos.PolicyAgent'>
|
|
<method name='Ask'>
|
|
<arg type='s' name='source' direction='in'/>
|
|
<arg type='s' name='service_name' direction='in'/>
|
|
<arg type='as' name='targets' direction='in'/>
|
|
<arg type='s' name='default_target' direction='in'/>
|
|
<arg type='a{ss}' name='icons' direction='in'/>
|
|
<arg type='s' name='response' direction='out'/>
|
|
</method>
|
|
</interface>
|
|
</node>
|
|
"""
|
|
|
|
def Ask(self, source, service_name, targets, default_target,
|
|
icons):
|
|
entries_info = {}
|
|
for target in targets:
|
|
entries_info[target] = {}
|
|
entries_info[target]['icon'] = icons.get(target, None)
|
|
|
|
response = qubespolicy.rpcconfirmation.confirm_rpc(
|
|
entries_info, source, service_name,
|
|
targets, default_target or None)
|
|
return response or ''
|
|
|
|
|
|
def main():
|
|
loop = GLib.MainLoop()
|
|
bus = pydbus.SystemBus()
|
|
obj = PolicyAgent()
|
|
bus.publish('org.qubesos.PolicyAgent', obj)
|
|
loop.run()
|
|
|
|
|
|
if __name__ == '__main__':
|
|
main()
|