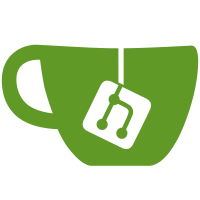
We assume that the template's root fs already has the following symlinks: /home -> /rw/home /usr/local -> /rw/usrlocal
113 linhas
2.7 KiB
Bash
Ficheiro executável
113 linhas
2.7 KiB
Bash
Ficheiro executável
#!/bin/sh
|
|
#
|
|
# chkconfig: 345 90 90
|
|
# description: Executes Qubes core scripts at VM boot
|
|
#
|
|
# Source function library.
|
|
. /etc/rc.d/init.d/functions
|
|
|
|
possibly_run_save_script()
|
|
{
|
|
ENCODED_SCRIPT=$(xenstore-read qubes_save_script)
|
|
if [ -z "$ENCODED_SCRIPT" ] ; then return ; fi
|
|
echo $ENCODED_SCRIPT|perl -e 'use MIME::Base64 qw(decode_base64); local($/) = undef;print decode_base64(<STDIN>)' >/tmp/qubes_save_script
|
|
chmod 755 /tmp/qubes_save_script
|
|
Xorg -config /etc/X11/xorg-preload-apps.conf :0 &
|
|
sleep 2
|
|
DISPLAY=:0 su - user -c /tmp/qubes_save_script
|
|
killall Xorg
|
|
}
|
|
|
|
start()
|
|
{
|
|
echo -n $"Executing Qubes Core scripts:"
|
|
|
|
if ! [ -x /usr/bin/xenstore-read ] ; then
|
|
echo "ERROR: /usr/bin/xenstore-read not found!"
|
|
exit 1
|
|
fi
|
|
if xenstore-read qubes_save_request 2>/dev/null ; then
|
|
ln -sf /home_volatile /home
|
|
possibly_run_save_script
|
|
touch /etc/this_is_dvm
|
|
dmesg -c >/dev/null
|
|
free | grep Mem: |
|
|
(read a b c d ; xenstore-write device/qubes_used_mem $c)
|
|
# we're still running in DispVM template
|
|
echo "Waiting for save/restore..."
|
|
# ... wait until qubes_restore.c (in Dom0) recreates VM-specific keys
|
|
while ! xenstore-read qubes_restore_complete 2>/dev/null ; do
|
|
usleep 10
|
|
done
|
|
echo Back to life.
|
|
fi
|
|
|
|
name=$(/usr/bin/xenstore-read name)
|
|
if ! [ -f /etc/this_is_dvm ] ; then
|
|
# we don't want to set hostname for DispVM
|
|
# because it makes some of the pre-created dotfiles invalid (e.g. .kde/cache-<hostname>)
|
|
# (let's be frank: nobody's gonna use xterm on DispVM)
|
|
hostname $name
|
|
fi
|
|
|
|
ip=$(/usr/bin/xenstore-read qubes_ip)
|
|
netmask=$(/usr/bin/xenstore-read qubes_netmask)
|
|
gateway=$(/usr/bin/xenstore-read qubes_gateway)
|
|
secondary_dns=$(/usr/bin/xenstore-read qubes_secondary_dns)
|
|
if [ x$ip != x ]; then
|
|
/sbin/ifconfig eth0 $ip netmask 255.255.255.255 up
|
|
/sbin/route add default dev eth0
|
|
echo "nameserver $gateway" > /etc/resolv.conf
|
|
echo "nameserver $secondary_dns" >> /etc/resolv.conf
|
|
fi
|
|
|
|
if [ -e /dev/xvdb ] ; then
|
|
mount /rw
|
|
|
|
if ! [ -d /rw/home ] ; then
|
|
echo
|
|
echo "--> Virgin boot of the VM: Linking /home to /rw/home"
|
|
|
|
mkdir -p /rw/config
|
|
touch /rw/config/rc.local
|
|
|
|
mkdir -p /rw/home
|
|
cp -a /home.orig/user /home
|
|
|
|
mkdir -p /rw/usrlocal
|
|
cp -a /usr/local.orig/* /usr/local
|
|
|
|
touch /var/lib/qubes/first_boot_completed
|
|
fi
|
|
fi
|
|
|
|
MEM_CHANGE_THRESHOLD_KB=30000
|
|
MEMINFO_DELAY_USEC=100000
|
|
/usr/lib/qubes/meminfo-writer $MEM_CHANGE_THRESHOLD_KB $MEMINFO_DELAY_USEC &
|
|
|
|
[ -x /rw/config/rc.local ] && /rw/config/rc.local
|
|
success
|
|
echo ""
|
|
return 0
|
|
}
|
|
|
|
stop()
|
|
{
|
|
return 0
|
|
}
|
|
|
|
case "$1" in
|
|
start)
|
|
start
|
|
;;
|
|
stop)
|
|
stop
|
|
;;
|
|
*)
|
|
echo $"Usage: $0 {start|stop}"
|
|
exit 3
|
|
;;
|
|
esac
|
|
|
|
exit $RETVAL
|