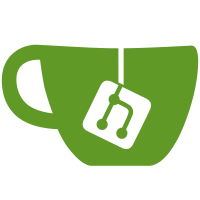
Configure package manager to use 127.0.0.1:8082 as proxy instead of "magic" IP intercepted later. The listen on this port and whenever new connection arrives, spawn qubes.UpdatesProxy service call (to default target domain - subject to configuration in dom0) and connect its stdin/out to the local TCP connection. This part use systemd.socket unit in case of systemd, and ncat --exec otherwise. On the other end - in target domain - simply pass stdin/out to updates proxy (tinyproxy) running locally. It's important to _not_ configure the same VM to both be updates proxy and use it. In practice such configuration makes little sense - if VM can access network (which is required to run updates proxy), package manager can use it directly. Even if this network access is through some VPN/Tor. If a single VM would be configured as both proxy provider and proxy user, connection would loop back to itself. Because of this, proxy connection redirection (to qrexec service) is disabled when the same VM also run updates proxy. Fixes QubesOS/qubes-issues#1854
141 lines
4.5 KiB
Bash
Executable File
141 lines
4.5 KiB
Bash
Executable File
#!/bin/bash
|
|
#
|
|
# The Qubes OS Project, http://www.qubes-os.org
|
|
#
|
|
# Copyright (C) 2015 Marek Marczykowski-Górecki
|
|
# <marmarek@invisiblethingslab.com>
|
|
#
|
|
# This program is free software; you can redistribute it and/or
|
|
# modify it under the terms of the GNU General Public License
|
|
# as published by the Free Software Foundation; either version 2
|
|
# of the License, or (at your option) any later version.
|
|
#
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with this program; if not, write to the Free Software
|
|
# Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
#
|
|
#
|
|
|
|
# Source Qubes library.
|
|
. /usr/lib/qubes/init/functions
|
|
|
|
BEGIN_MARKER="### QUBES BEGIN ###"
|
|
END_MARKER="### QUBES END ###"
|
|
|
|
set -e
|
|
|
|
### helper functions begin ###
|
|
|
|
# set proxy in given config file
|
|
update_conf() {
|
|
local CONF_PATH="$1"
|
|
local CONF_OPTIONS="$2"
|
|
|
|
# Ensure that Qubes conf markers are present in the file
|
|
if ! grep -q "$BEGIN_MARKER" $CONF_PATH; then
|
|
if grep -q "$END_MARKER" $CONF_PATH; then
|
|
echo "ERROR: found QUBES END marker but not QUBES BEGIN in ${CONF_PATH}" >&2
|
|
echo "Fix the file by either removing both of them, or adding missing back and retry" >&2
|
|
exit 1
|
|
fi
|
|
cp $CONF_PATH ${CONF_PATH}.qubes-orig
|
|
echo "$BEGIN_MARKER" >> $CONF_PATH
|
|
echo "$END_MARKER" >> $CONF_PATH
|
|
elif ! grep -q "$END_MARKER" $CONF_PATH; then
|
|
echo "ERROR: found QUBES BEGIN marker but not QUBES END in ${CONF_PATH}" >&2
|
|
echo "Fix the file by either removing both of them, or adding missing back and retry" >&2
|
|
exit 1
|
|
fi
|
|
|
|
# Prepare config block
|
|
local tmpfile=`mktemp`
|
|
cat > ${tmpfile} <<EOF
|
|
# This part of configuration, until QUBES END, is automatically generated by
|
|
# $0. All changes here will be overriden.
|
|
# If you want to override any option set here, set it again to desired value,
|
|
# below this section
|
|
$CONF_OPTIONS
|
|
EOF
|
|
|
|
# And insert it between the markers
|
|
sed -i -e "/^$BEGIN_MARKER$/,/^$END_MARKER$/{
|
|
/^$END_MARKER$/b
|
|
/^$BEGIN_MARKER$/!d
|
|
r ${tmpfile}
|
|
}" ${CONF_PATH}
|
|
rm -f ${tmpfile}
|
|
}
|
|
|
|
### helper functions end
|
|
|
|
# Determine whether the proxy should be used
|
|
if qsvc yum-proxy-setup || qsvc updates-proxy-setup ; then
|
|
PROXY_ADDR="http://127.0.0.1:8082/"
|
|
PROXY_CONF_ENTRY="proxy=$PROXY_ADDR"
|
|
else
|
|
PROXY_ADDR=""
|
|
# do not proxy at all (for example dnf.conf doesn't tolerate empty entry)
|
|
PROXY_CONF_ENTRY=""
|
|
fi
|
|
|
|
# For programs supporting .d style configs, it's simple
|
|
if [ -d /etc/apt/apt.conf.d ]; then
|
|
if [ -n "$PROXY_ADDR" ]; then
|
|
cat > /etc/apt/apt.conf.d/01qubes-proxy <<EOF
|
|
### This file is automatically generated by Qubes ($0 script).
|
|
### All modifications here will be lost.
|
|
### If you want to override some of this settings, create another file under
|
|
### /etc/apt/apt.conf.d.
|
|
|
|
Acquire::http::Proxy "$PROXY_ADDR";
|
|
EOF
|
|
else
|
|
rm -f /etc/apt/apt.conf.d/01qubes-proxy
|
|
fi
|
|
fi
|
|
|
|
# Yum at least supports including an individual config files
|
|
if [ -d /etc/yum.conf.d ]; then
|
|
cat > /etc/yum.conf.d/qubes-proxy.conf <<EOF
|
|
### This file is automatically generated by Qubes ($0 script).
|
|
### All modifications here will be lost.
|
|
### If you want to override some of this settings, add them in /etc/yum.conf
|
|
### below a "include=/etc/yum.conf.d/qubes-proxy.conf" line.
|
|
|
|
$PROXY_CONF_ENTRY
|
|
EOF
|
|
fi
|
|
|
|
# Pacman (archlinux) also
|
|
if [ -d /etc/pacman.d ]; then
|
|
if [ -n "$PROXY_ADDR" ]; then
|
|
cat > /etc/pacman.d/01-qubes-proxy.conf <<EOF
|
|
### This file is automatically generated by Qubes ($0 script).
|
|
### All modifications here will be lost.
|
|
### If you want to override some of this settings, create another file under
|
|
### /etc/pacman.d
|
|
|
|
XferCommand = http_proxy=$PROXY_ADDR /usr/bin/curl -C - -f %u > %o
|
|
EOF
|
|
else
|
|
rm -r /etc/pacman.d/01-qubes-proxy.conf
|
|
fi
|
|
fi
|
|
|
|
# DNF configuration doesn't support including other files
|
|
# https://bugzilla.redhat.com/show_bug.cgi?id=1352234
|
|
if [ -e /etc/dnf/dnf.conf ]; then
|
|
update_conf /etc/dnf/dnf.conf "$PROXY_CONF_ENTRY"
|
|
fi
|
|
|
|
# The same goes for PackageKit...
|
|
# https://bugs.freedesktop.org/show_bug.cgi?id=96788
|
|
if [ -e /etc/PackageKit/PackageKit.conf ]; then
|
|
update_conf /etc/PackageKit/PackageKit.conf "ProxyHTTP=$PROXY_ADDR"
|
|
fi
|